Developer Guide to Document Authoring with Edge Delivery Services. Part 9
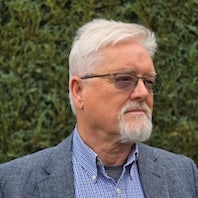
Comprehensive Guide to AEM Edge Delivery Services Development
Previously
My last article was Part 8 - Using AI with Edge Delivery Services
Part 9: Detailed Guide for Developer Setup
1. Detailed Setup Process
1.1 GitHub Repository Setup
1. Go to GitHub and create a new repository.
2. Use the [AEM Boilerplate](https://github.com/adobe/aem-boilerplate) as a template:
- Click "Use this template"
- Name your repository
- Choose public or private visibility
3. Clone the repository to your local machine:
git clone https://github.com/your-username/your-repo-name.git
cd your-repo-name
1.2 AEM CLI Installation and Usage
1. Install the AEM CLI globally:
npm install -g @adobe/aem-cli
2. Key AEM CLI commands:
- aem up: Start the local development server
- aem import: Import pages from an existing website
- aem help: Get help
1.3 Content Source Configuration
Google Drive Setup:
1. Create a new folder in Google Drive
2. Share the folder with helix@adobe.com with edit permissions
3. Copy the folder ID from the URL
Or SharePoint Setup:
1. Create a new SharePoint site or use an existing one
2. Create a document library for your project
3. Share the document library with helix@adobe.com with edit permissions
1.4 Configuring fstab.yaml
Create fstab.yaml in your project root:
mountpoints:
/: https://drive.google.com/drive/folders/YOUR_FOLDER_ID
For SharePoint:
mountpoints:
/: https://{tenant}.sharepoint.com/sites/{site}/{document-library}
1.5 AEM Sidekick Extension Setup
1. Install the extension from [AEM Sidekick](https://www.aem.live/tools/sidekick/)
2. Configure the extension:
- Open the extension options
- Add your project URL (e.g., https://main--your-repo--your-org.hlx.page/)
2. Project Structure and Key Files
├── blocks/
│ └── header/
│ ├── header.css
│ └── header.js
├── icons/
├── scripts/
│ ├── aem.js
│ └── scripts.js
├── styles/
│ ├── styles.css
│ └── lazy-styles.css
├── tools/
│ └── sidekick/
│ └── config.json
├── 404.html
├── 500.html
├── head.html
├── footer.html
├── helix-query.yaml
└── fstab.yaml
Key files:
- scripts/scripts.js: Main JavaScript file
- styles/styles.css: Main CSS file
- head.html: Custom <head> content
- helix-query.yaml: Configure content indexing
- tools/sidekick/config.json: Sidekick configuration
3. Detailed Development Workflow
3.1 Creating and Decorating Blocks
1. Create a new folder in /blocks/your-block-name/
2. Add your-block-name.css and your-block-name.js
3. In the JS file:
export default async function decorate(block) {
// DOM manipulation
const wrapper = document.createElement('div');
wrapper.className = 'your-block-name-wrapper';
// Replace block content
block.textContent = '';
block.appendChild(wrapper);
// Add interactivity
wrapper.addEventListener('click', () => {
console.log('Block clicked!');
});
// Async operations (if needed)
try {
const response = await fetch('/api/data.json');
if (!response.ok) throw new Error('Network response was not ok');
const data = await response.json();
// Use data to update block
} catch (error) {
console.error('Failed to fetch data:', error);
}
}
3.2 Styling Best Practices
1. Use CSS variables for theming:
:root {
--primary-color: #007bff;
--secondary-color: #6c757d;
}
2. Implement responsive design:
.your-block-name {
display: flex;
flex-direction: column;
}
@media (min-width: 768px) {
.your-block-name {
flex-direction: row;
}
}
3.3 Content Authoring
1. Open Google Docs or Microsoft Word
2. Structure content using headings (H1, H2, etc.)
3. Use tables to define blocks:
4. Add metadata at the end of the document:
3.4 Advanced Features
Auto-blocking
AEM can automatically create blocks based on content structure. Example:
function buildAutoBlocks(main) {
const h1 = main.querySelector('h1');
if (h1) {
const picture = h1.previousElementSibling?.querySelector('picture');
if (picture) {
const section = picture.closest('.section');
if (section) {
section.classList.add('hero-container');
const wrapper = document.createElement('div');
wrapper.className = 'hero';
wrapper.append(picture, h1);
section.prepend(wrapper);
}
}
}
}
Using Spreadsheets for Dynamic Content
1. Create a spreadsheet in your content folder
2. Structure data with headers in the first row
3. Access data in your block:
async function fetchData() {
const response = await fetch('/your-spreadsheet.json');
if (!response.ok) throw new Error('Failed to fetch data');
return response.json();
}
export default async function decorate(block) {
try {
const data = await fetchData();
// Use data to populate block
data.forEach(item => {
const element = document.createElement('div');
element.textContent = item.columnName;
block.appendChild(element);
});
} catch (error) {
console.error('Error:', error);
}
}
4. Performance Optimization
1. Implement lazy loading for images:
import { createOptimizedPicture } from '../scripts/aem.js';
// In your block's decorate function:
const images = block.querySelectorAll('img');
images.forEach(img => {
const optimizedPicture = createOptimizedPicture(img.src, img.alt, false, [{ width: '750' }]);
img.parentElement.replaceChild(optimizedPicture, img);
});
2. Use the E-L-D (Eager-Lazy-Delayed) loading pattern:
- Eager: Critical content and above-the-fold elements
- Lazy: Content that can be loaded as the user scrolls
- Delayed: Non-essential third-party scripts or tracking
3. Optimize CSS delivery:
- Critical CSS in styles.css
- Non-critical CSS in lazy-styles.css
4. Minimize JavaScript execution time:
- Use efficient DOM manipulation techniques
- Debounce or throttle event listeners for performance-intensive operations
5. Testing and Debugging
1. Use browser developer tools to inspect and debug your blocks
2. Implement unit tests for your JavaScript functions
3. Use Lighthouse in Chrome DevTools to audit performance, accessibility, and SEO
4. Test on multiple devices and browsers to ensure responsiveness and cross-browser compatibility
6. Deployment and Publishing
1. Commit and push your changes to GitHub
2. AEM will automatically build and deploy your changes to the preview environment
3. Use the AEM Sidekick to publish content changes
4. For production deployment, configure your CDN to point to your AEM live environment
7. Monitoring and Analytics
1. Implement Real User Monitoring (RUM) to track performance metrics
2. Use Adobe Analytics or Google Analytics for user behavior tracking
3. Monitor your site's health using tools like Adobe Experience Manager's built-in monitoring capabilities
Last Words
Use AEM's extensive documentation, community resources, and support channels throughout development. Leverage the Block Party for community-contributed components and stay updated with the latest AEM features and best practices.
Remember to follow AEM's performance guidelines, content modelling best practices, and security recommendations to ensure a robust, efficient, and secure website. If you need to handle user input or data submission, consider implementing custom solutions using Edge Functions or integrating with external services that comply with your project's requirements and data handling policies.
Following this comprehensive guide, you'll be well-equipped to develop sophisticated, high-performance websites using AEM Edge Delivery Services. Always refer to the official AEM documentation and stay updated with the latest best practices and features.
https://aem.live
Thanks for reading.
Digital Domain Technologies provides expert Adobe Experience Manager (AEM) consultancy. We have collaborated with some of the world’s leading brands across various AEM platforms, including AEM Cloud, on-premise solutions, Adobe Managed Services, and Edge Delivery Services. Our portfolio includes partnerships with prominent companies such as Twitter (now X), EE, Nissan/Renault Alliance Ford, Jaguar Land Rover, McLaren Sports Cars, Hyundai Genesis, and many others.
Related Articles